In the ever-evolving world of programming, LeetCode stands as a beacon for budding and seasoned coders alike. Among the myriad challenges it offers, one intriguing problem is "find the dominant element leetcode." This task not only tests one's understanding of algorithms and data structures but also challenges problem-solving skills. With the rise of competitive programming, mastering such problems is more crucial than ever. The "dominant element" problem asks programmers to identify the element in an array that appears more than ⌊n/2⌋ times, where n is the size of the array, a classic problem that has its roots in several real-world applications.
Understanding the "find the dominant element leetcode" problem requires a deep dive into algorithm optimization and complexity analysis. Efficiently solving this problem can lead to better performance in code execution, especially in environments where processing speed and memory usage are of paramount importance. From beginners to advanced coders, grasping the intricacies of this problem can open doors to more sophisticated algorithmic challenges. The problem is often approached using techniques such as sorting, hash maps, and the Boyer-Moore Voting Algorithm, each offering unique insights into algorithm efficiency and design patterns.
As we delve deeper into this topic, this article aims to provide a comprehensive guide to understanding and solving the "find the dominant element leetcode" problem. We will explore various methods, analyze their time and space complexities, and discuss the practical applications of these techniques. Whether you're preparing for coding interviews or looking to enhance your algorithmic skills, this guide will serve as an invaluable resource. We'll also address common questions and misconceptions, ensuring a holistic understanding of the problem and its solutions. So, let's embark on this journey to demystify one of LeetCode's classic challenges.
Table of Contents
- Understanding the Problem
- Naive Approach to Solving the Problem
- Using Sorting to Find the Dominant Element
- Hash Map Method
- Boyer-Moore Voting Algorithm
- Time and Space Complexity Analysis
- Real-World Applications
- Common Misconceptions
- Frequently Asked Questions
- Conclusion
Understanding the Problem
The "find the dominant element leetcode" problem is a classic example of the majority element problem, where the goal is to identify the element that appears more than half the time in a given list. This challenge is not only about finding an element but also about doing so efficiently. This problem usually involves an array of integers, and the task is to determine which number, if any, appears more than ⌊n/2⌋ times.
The significance of this problem extends beyond coding exercises. In practical scenarios, identifying a majority element could apply to data analytics, where understanding dominant trends or behaviors is crucial. For instance, in voting systems, it's vital to identify a candidate who receives more than half of the votes, ensuring a clear majority.
To solve this problem, one must consider both time complexity, which measures how the runtime grows with input size, and space complexity, which considers the amount of memory used. For instance, a brute force approach might involve checking each element's frequency, but this could become inefficient with large datasets. Thus, understanding the nuances of the problem and the constraints provided by LeetCode is essential to devising an optimal solution.
Naive Approach to Solving the Problem
At first glance, the most straightforward way to tackle the "find the dominant element leetcode" problem is through a brute force method. This involves iterating through the array and counting the occurrence of each element. While conceptually simple, this approach quickly becomes inefficient as it requires two nested loops: one to pick an element and another to count its occurrences. The time complexity of this method is O(n^2), which is impractical for large datasets.
Despite its inefficiency, the brute force approach serves as a good starting point for understanding the problem. It lays the foundation for more sophisticated methods by highlighting the need to reduce redundant calculations. Further, while not suitable for competitive programming or real-time applications, this method can be useful for educational purposes or when dealing with small datasets.
Using Sorting to Find the Dominant Element
One of the more efficient methods to tackle the "find the dominant element leetcode" problem is by using sorting. By sorting the array, the majority element, if it exists, must occupy the middle position. This is because if an element appears more than half the time, it will dominate the central portion of a sorted array.
The sorting approach reduces the time complexity to O(n log n) due to the sorting operation. After sorting, a single pass through the array is sufficient to confirm the majority element. However, this method has a space complexity of O(1) if the sorting is done in place, making it a viable option for many practical applications where memory usage is a concern.
While sorting provides an improvement over the brute force method, it still might not be the most optimal solution for situations with stringent time constraints. Nonetheless, it is a significant step forward in understanding how reordering data can simplify the problem-solving process.
Hash Map Method
The hash map method offers an even more efficient approach to solving the "find the dominant element leetcode" problem. By utilizing a hash map, we can store the frequency of each element in the array, allowing us to determine the majority element in linear time, O(n).
This method involves iterating through the array once to populate the hash map and then iterating through the map to find the element with the highest count. The space complexity is O(n) due to the storage requirements of the hash map. While this method is efficient, it's important to consider scenarios with memory limitations, where a different approach might be necessary.
The hash map method is particularly useful in applications where data retrieval speed is critical. It demonstrates the power of hash tables in providing constant time complexity for data access, making it a staple in the toolkit of any programmer dealing with large datasets.
Boyer-Moore Voting Algorithm
The Boyer-Moore Voting Algorithm stands as a pinnacle of efficiency for solving the "find the dominant element leetcode" problem. This algorithm operates in linear time, O(n), and constant space, O(1), making it the most optimal solution for this problem.
The Boyer-Moore Voting Algorithm works by maintaining a candidate for the majority element and a counter that tracks the candidate's validity. As we iterate through the array, the counter increases when the current element matches the candidate and decreases otherwise. If the counter reaches zero, we select a new candidate. By the end of the array, the candidate is the majority element, if it exists.
This algorithm is not only efficient but also elegant, embodying the principle of optimal substructure. It is particularly effective in environments where both time and space are constrained, and it serves as a prime example of algorithmic ingenuity in computer science.
Time and Space Complexity Analysis
An in-depth understanding of time and space complexity is crucial when solving the "find the dominant element leetcode" problem. Each method discussed offers different trade-offs between time and space, making it necessary to choose the right one based on the specific context of the problem.
The brute force method, while simple, has a time complexity of O(n^2) and space complexity of O(1), making it unsuitable for large datasets. The sorting method improves the time complexity to O(n log n) with a space complexity of O(1), offering a balance between time and memory usage.
The hash map method further reduces the time complexity to O(n) but at the cost of space, requiring O(n) additional memory. Conversely, the Boyer-Moore Voting Algorithm provides the best of both worlds with a time complexity of O(n) and space complexity of O(1).
Understanding these complexities not only aids in solving the problem efficiently but also in making informed decisions about the suitability of each method in different scenarios.
Real-World Applications
The "find the dominant element leetcode" problem, while an academic exercise, has significant real-world implications. In data analytics, identifying the majority element can help in understanding dominant trends or behaviors. For example, in social media analytics, it can be used to determine the most popular topic or hashtag within a set time frame.
In voting systems, accurately identifying a majority candidate is crucial for ensuring fair election results. Furthermore, in distributed computing, finding the dominant element can aid in consensus algorithms where agreement among nodes is necessary.
These applications highlight the importance of understanding and efficiently solving the "find the dominant element leetcode" problem. By mastering this challenge, one can apply these skills to a wide range of practical scenarios, enhancing the ability to analyze and interpret large datasets.
Common Misconceptions
Despite its apparent simplicity, the "find the dominant element leetcode" problem is often misunderstood. One common misconception is that a majority element always exists, which is not the case. It is essential to verify if the candidate actually appears more than ⌊n/2⌋ times.
Another misunderstanding is equating the presence of duplicates with the existence of a majority element. While duplicates may exist, they do not necessarily imply a majority. It's crucial to accurately count occurrences to confirm a true majority.
Lastly, some may assume that the most frequent element is always the majority. However, unless it meets the ⌊n/2⌋ threshold, it cannot be considered as such. These misconceptions underscore the importance of a thorough understanding of the problem's requirements and constraints.
Frequently Asked Questions
What is the dominant element in an array?
The dominant element, also known as the majority element, is the element that appears more than ⌊n/2⌋ times in an array, where n is the size of the array.
Can there be more than one dominant element?
No, by definition, there can only be one dominant element in an array, as it must appear more than half the time.
What is the best algorithm to find the dominant element?
The Boyer-Moore Voting Algorithm is considered the most efficient, operating in O(n) time and O(1) space.
How do you handle arrays without a majority element?
If no element meets the ⌊n/2⌋ threshold, then the array does not have a majority element. This must be accounted for in the solution.
Is sorting always necessary to find the dominant element?
No, sorting is one method but not necessary. Other techniques like the Boyer-Moore Voting Algorithm provide solutions without sorting.
Does the presence of duplicates affect the majority element?
Duplicates alone do not imply a majority. The element must appear more than ⌊n/2⌋ times to be considered the majority.
Conclusion
Mastering the "find the dominant element leetcode" problem not only prepares one for coding interviews and competitive programming but also enhances problem-solving skills applicable to real-world scenarios. By exploring various methods from brute force to the Boyer-Moore Voting Algorithm, one gains a comprehensive understanding of algorithm efficiency and optimization. As we have seen, each method offers unique insights and trade-offs, underscoring the importance of selecting the right approach based on specific requirements. With this knowledge, programmers can confidently tackle similar challenges, ensuring both accuracy and efficiency in their solutions.
For those interested in delving deeper into algorithmic challenges, resources like GeeksforGeeks provide extensive tutorials and problem sets to further enhance one's coding prowess.
You Might Also Like
Unveiling The Versatility And Elegance Of Women's Grey TopsThe Fascinating World Of Gorillaz Freaks: An In-Depth Exploration
Discover The Samsung 65Q72D: A Comprehensive Exploration Of Its Features And Benefits
Mastering The Art Of Relocation For Your Dream Job: A Comprehensive Guide
Exploring The World Of Football Card Packs: A Collector's Dream
Article Recommendations
- Monopoly Cars
- Two Front Door House
- 22 Tcm
- Delete Hulu Watch History
- Blue Hanfu
- Best Indoor Plants For Asthma Uk
- Harvard Rejection
- Wicker Outdoor Furniture
- Winter Essentials Woman
- Foot Booties Peel

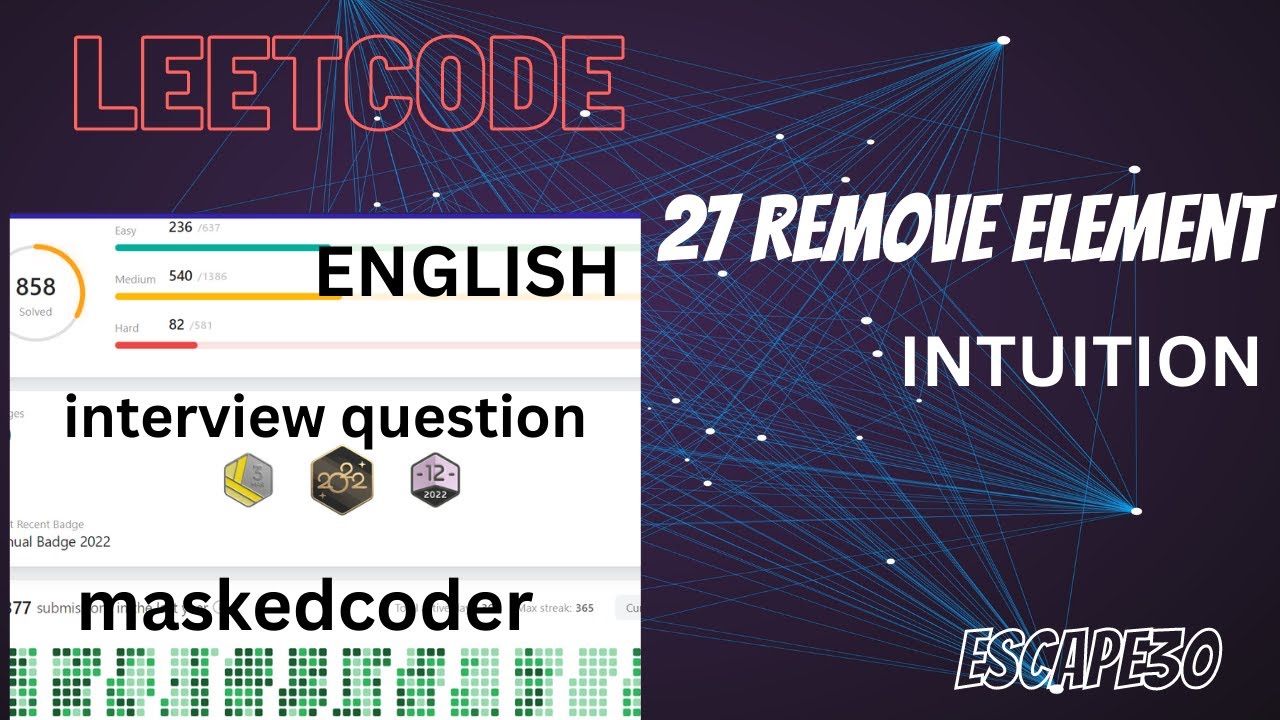